In this post I am going to share my workflow and best practices for using Visual Studio Code as a development environment for ROS.
Important note: you need to open the ROS work space with vs code, not only your specific package. Otherwise this guide will not work for you.
Plugins
I will start by specifying the plugins I use in my environment in no particular order.
C/C++
This is the standard C/C++ plugin for utilizing Microsoft’s IntelliSense code completion. It requires information about the dependencies that are used in the project. Thankfully, CMake is able to provide this information because it is our build system and finds the dependencies in the first place. It provides this information by exporting a file called compile_commands.json which the C/C++ plugin can work with. You can also specify things like your compiler type (GNU / Clang) or the C++ version that the project is built against. All of this information is put in the file c_cpp_properties.json in the .vscode directory of your ROS workspace:
{
"configurations": [
{
"name": "Linux",
"includePath": [
"${workspaceFolder}/**"
],
"defines": [],
"compilerPath": "/usr/bin/gcc",
"cStandard": "c11",
"cppStandard": "c++14",
"intelliSenseMode": "gcc-x64",
"compileCommands": "${workspaceFolder}/build/compile_commands.json"
}
],
"version": 4
}
I will explain how the compile_commands.json file is generated with catkin_make / cmake in the tasks section below.
CMake
The CMake plugin is useful for auto-completion when modifying the CMakeLists.txt file of one of your packages. It shouldn’t be confused with the plugin ‘CMake Tools’ which automates the CMake configuration and build process within vs code. No configuration is needed for this plugin.
ROS
The ROS plugin provides common ROS tasks like package creation, ROS master monitoring and most important for me .msg and .urdf file syntax highlighting.
Clang-Format
This formatter is useful for automatically formatting C++ ROS nodes once they are saved. Clang-format needs to be installed on your Ubuntu system:
sudo apt-get install clang-format
Also, go to the settings by typing ‘Ctrl + ,’ and search for ‘editor.formatOnSave’ and set it to true. Otherwise the files need to be formatted with manual commands.
vscode-icons
This plugin swaps the default set of icons for visually more distinct icons so that .launch, .xml and .cpp files can be distinguished from each other when using the file explorer sidebar on the left. Once it is installed press ‘Ctrl + Shift + P’ and type ‘activate icons’ to activate it.
Visual Studio Code tasks
Visual Studio Code provides a powerful interface for automating frequent tasks. In order to use it in a more comfortable manner though we will set keybindings for our most common tasks: catkin_make and running arbitrary ROS nodes or launch files. Go to File -> Preferences -> Keyboard Shortcuts and set the following keybindings:
'Run Build Task': 'Ctrl + Shift + B'
'Run Task': 'Ctrl + Shift + 3'
After that, create the the file tasks.json in the directory .vscode and paste the followiong example content in there:
{
"version": "2.0.0",
"tasks": [
{
"label": "catkin_make",
"type": "shell",
"command": "catkin_make",
"args": [
"-j4",
"-DCMAKE_BUILD_TYPE=Release",
"-DCMAKE_EXPORT_COMPILE_COMMANDS=1",
"-DCMAKE_CXX_STANDARD=14"
],
"problemMatcher": [],
"group": {
"kind": "build",
"isDefault": true
}
},
{
"label": "panda_simulation",
"type": "shell",
"command": "roslaunch panda_simulation simulation.launch",
"problemMatcher": [],
"group": {
"kind": "test",
"isDefault": true
}
}
]
}
The first task is for building the work space and can be executed with the previously defined shortcut ‘Ctrl + Shift + B’. The second task is an example for launching a launch file and can be executed with the shortcut ‘Ctrl + Shift + 3’ where it can be selected with the arrow keys.
- You can add more CMake arguments by extending the args array
- The problem matcher would specify the task output interpreter. We don’t use it. By specifying an empty array we avoid a prompt that asks for the problem matcher every time the task is executed
- Within the group tag the task type is specified
The compile_commands.json file that I mentioned earlier is generated by CMake if the argument CMAKE_EXPORT_COMPILE_COMMANDS=1 is passed to catkin_make. The C/C++ plugion will now be able to provide auto-completion when writing ROS nodes in C++.
Results
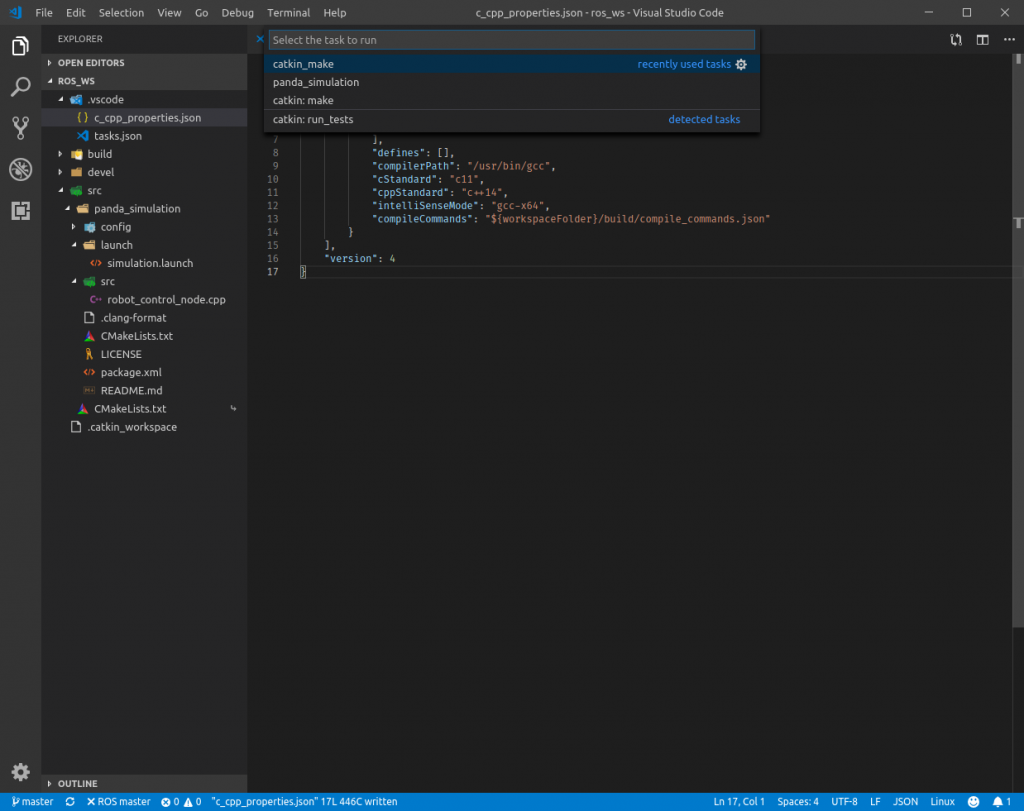
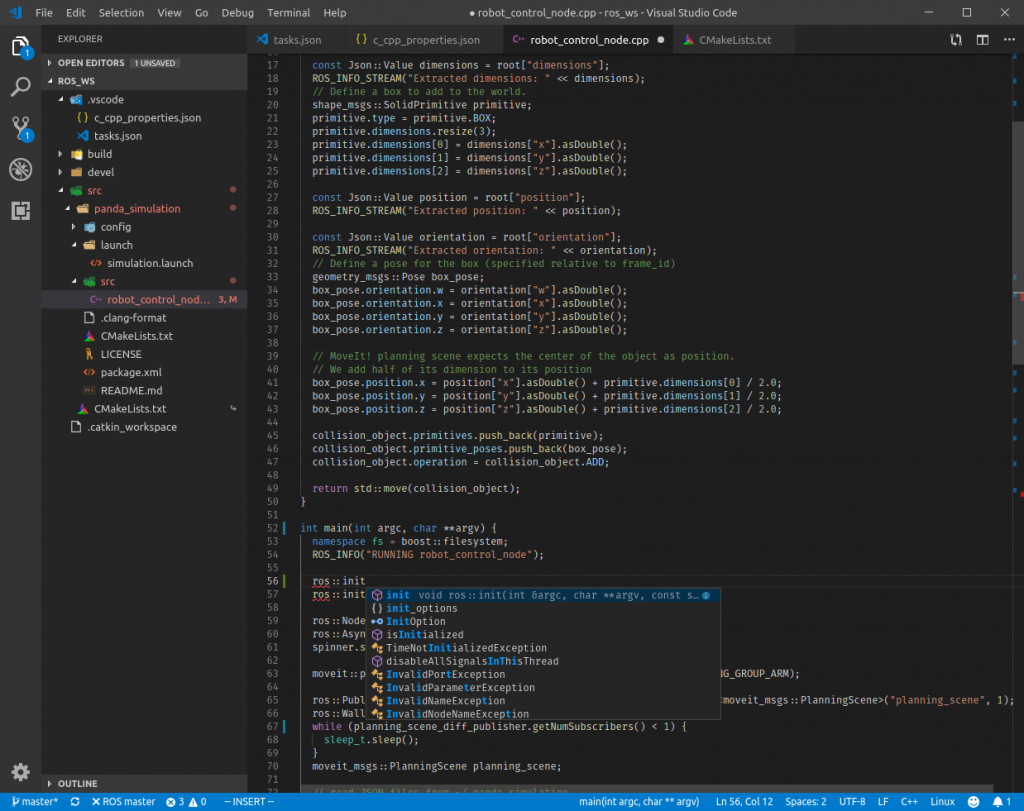
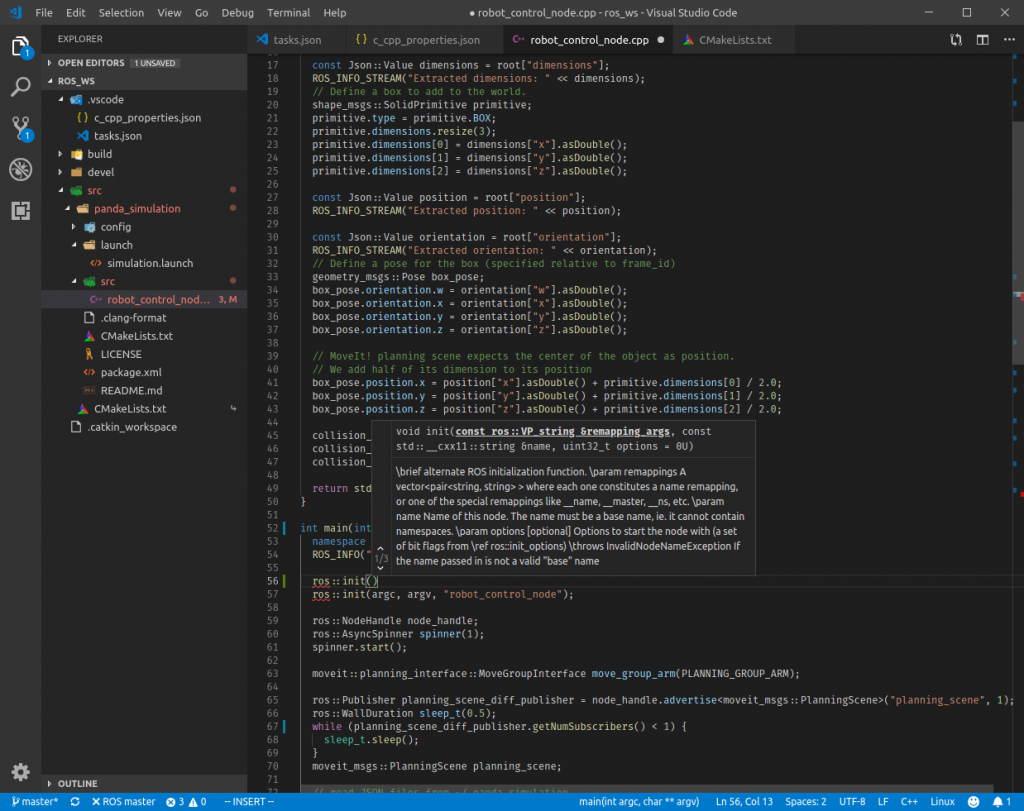
C++ auto completion writing a ROS node: method arguments and description
I have provided my .vscode directory as a GitHub repository.